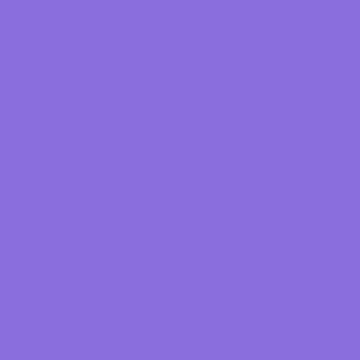
MultiSceneManager.cs
Assembly: CarterGames.MultiScene.Runtime
Namespace: CarterGames.Experimental.MultiScene
The multi scene manager is the main class to handle scene management with this asset. You should use this instead of the normal SceneManager
when it comes to loading scenes & checking if scenes are active.
Properties
ActiveSceneGroup
SceneGroup
→ Get
Gets the currently active scene group. This will update to whichever group is the latest loaded at runtime.
var group = MultiSceneManager.ActiveSceneGroup;
Events
BeforeScenesLoaded
void
→ Event
An event that raises just before the system loads a scene group.
MultiSceneManager.BeforeScenesLoaded.Add(MyMethod);
private void MyMethod()
{
.....
PostScenesLoaded
void
→ Event
An event that raises after the system had loaded a scene group & all interfaces have been called.
MultiSceneManager.PostScenesLoaded.Add(MyMethod);
private void MyMethod()
{
.....
OnSceneLoaded
string
→ Event
An event that raises after the system has loaded any scene, it passes through the name of the scene loaded as a param.
MultiSceneManager.OnSceneLoaded.Add(MyMethod);
private void MyMethod(string sceneName)
{
.....
OnSceneUnloaded
string
→ Event
An event that raises after the system has unloaded any scene, it passes through the name of the scene unloaded as a param.
MultiSceneManager.OnSceneUnloaded.Add(MyMethod);
private void MyMethod(string sceneName)
{
.....
OnSceneGroupLoaded
void
→ Event
An event that raises after the system has loaded a scene group, this event doesn’t pass through the scene group as a param. Use OnSceneGroupLoadedWithCtx for a passthrough.
MultiSceneManager.OnSceneGroupLoaded.Add(MyMethod);
private void MyMethod()
{
.....
OnSceneGroupLoadedWithCtx
SceneGroup
→ Event
An event that raises after the system has loaded a scene group, it passes the group loaded as a param.
MultiSceneManager.OnSceneGroupLoadedWithCtx.Add(MyMethod);
private void MyMethod(SceneGroup group)
{
.....
Methods
IsSceneInGroup
Method
string
→ The scene name to search for.
Returns
bool
→ Whether or not the scene is in the group.
A helper method to get whether or not a scene is in the currently loaded scene group.
private void MyMethod()
{
if (MultiSceneManager.IsSceneInGroup("MyScene")
{
// Logic here...
}
}
IsSceneInGroup
Method
SceneGroup
→ The scene group to search through.
Returns
bool
→ Whether or not the scene is in the group.
A helper method to get whether or not a scene is in the group entered.
SceneGroup group;
private void MyMethod()
{
if (MultiSceneManager.IsSceneInGroup(group, "MyScene")
{
// Logic here...
}
}
IsSceneLoaded
Method
string
→ The scene name to
Returns
bool
→ Whether or not the scene is in the group.
A helper method to get whether or not a scene is in the currently loaded.
private void MyMethod()
{
if (MultiSceneManager.IsSceneLoaded("MyScene")
{
// Logic here...
}
}
SetGroup
Method
SceneGroup
→ The scene group to load.
A helper method to sets the active group to the group entered.
private void MyMethod()
{
if (MultiSceneManager.IsSceneLoaded("MyScene")
{
// Logic here...
}
}
LoadScenes
Method
bool
→ Optional
→ Should the system reload scenes that are already loaded? Default: true
Loads the scene group currently set in the manager.
private void MyMethod()
{
MultiSceneManager.LoadScenes();
}
LoadScenes
Method
SceneGroup
→ The scene group to load.
bool
→ Optional
→ Should the system reload scenes that are already loaded? Default: true
Loads the scene group entered and sets it as the new active scene group.
SceneGroup group;
private void MyMethod()
{
MultiSceneManager.LoadScenes(group);
}
ReloadScenes
void
→ Method
Reloads the current active scene group.
SceneGroup group;
private void MyMethod()
{
MultiSceneManager.ReloadScenes();
}
UnloadAllActiveScenes
void
→ Method
Unloads all the scenes currently loaded & loads a blank placeholder scene.
private void MyMethod()
{
MultiSceneManager.UnloadAllActiveScenes();
}
UnloadAllAdditiveScenes
void
→ Method
Unloads all the additive
scenes currently loaded, but leaves the active (base
) scene still running.
private void MyMethod()
{
MultiSceneManager.UnloadAllAdditiveScenes();
}