Leaderboard Manager (2.1.0)
While it is a little delayed, the new rather long-awaited Leaderboard Manager update is here. So what’s new?
Summary of changes
Mechanically the asset is pretty much the same as the earlier 2.0.x versions of the asset but with some updated backend logic for future updates. A full rundown of the behind-the-scenes changes:
- A new saving system similar to the Save Manager’s setup with a JSON save setup instead of the legacy binary save file setup.
- Updated the structure of leaderboards to allow each entry to have its own unique identifier so you can reference an entry more easily.
There is a porting tool to update old saves to the new structure. More on this in a moment.
- New settings structure to match the rest of the assets released recently with per-user settings and a settings provider flow.
- Offline documentation is now complete instead of a simpler version. The online docs are also updated to match the rest of the assets using Notion as a host (easier to maintain in a pinch and has search built-in).
There is also a new editor to allow to viewing of existing leaderboards as well as a setup to port older saves to this new version, both in the editor and at runtime.
Editor
With the editor tab you can view all leaderboards currently in the save file. You can’t make new ones here as the API isn’t designed to have boards made in the editor, instead having them made at runtime. You can however delete board should you need to test the setup and view the entries to make sure they appear as expected which should help with debugging.
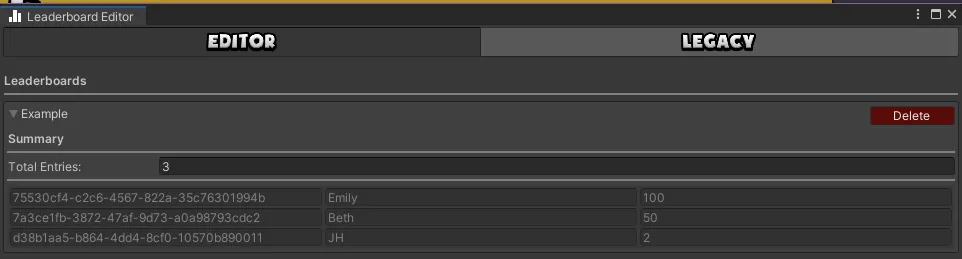
Porting data to 2.1.x from 2.0.x
As mentioned the new structure means any old data from the previous version will need updating. A full rundown is in the documentation for the asset. But basically, if you used the old setup to save the score as time by saving the score in seconds etc. As you can now save your score as into a time class instead. There isn’t any major work you need to do to achieve this, just define the board IDs and the type to convert them to and the asset should take care of the rest.
Note: you will need to convert boards even if they were just a score field.
An example below of a board called “Arcade” being converted to a scoreboard in the new version.
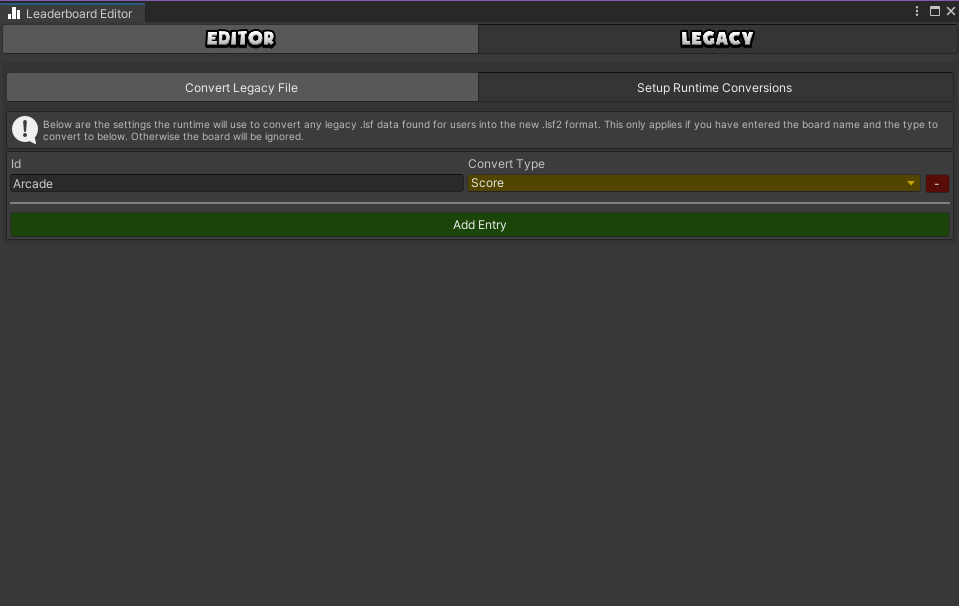
What’s next?
With this update done, I’m mainly focusing on support and getting back into my main side project for the year as I’ve had to take some time off of it to get this out. I do have a few game ideas that I may prototype at some point or at least write out some ideas for next year to work on proper. More on these when I have something to share.